How to Throw Exceptions in Python
Python now has around 19 million users, making it even more popular than JavaScript. For one thing, developers love Python exceptions and error handling.
If you’re new, it’s important to learn how to throw exceptions in Python. Any Python developer will tell you that it’s a very basic yet crucial skill to have.
The following guide will break down everything you need to know about throwing exceptions.
What It Means
Throwing an exception in Python involves an object referred to as an exception object. It includes information about errors such as their type and the program’s condition when the error happened.
Throwing an exception means creating an exception and then passing it onto the runtime system. If you don’t manage exceptions correctly in Python applications, the program might crash.
Thankfully, Python comes with a strong framework for managing errors to make things simple. It has a group of pre-defined exceptions and uses structured handling for exceptions.
Developers can identify what kind of error it is during run time and take action from there. Actions include taking a different path, utilizing default values, or using prompts for proper input.
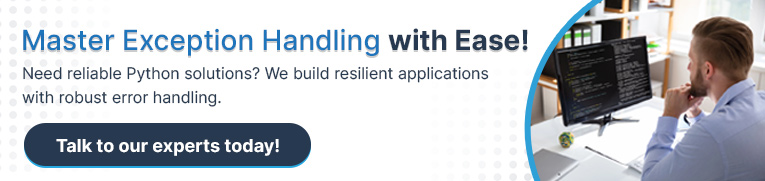
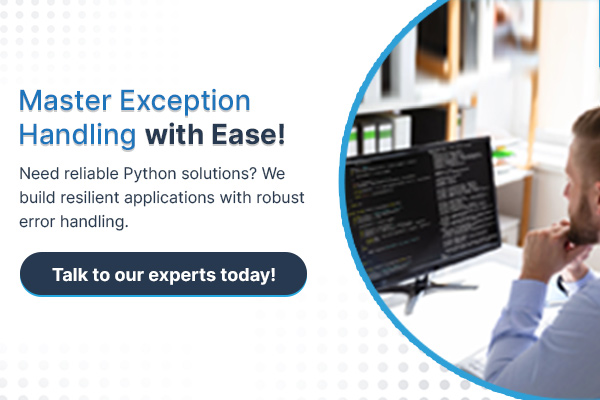
How to Throw Exceptions in Python
In some cases, you might need Python to throw a customized exception to handle certain errors. To accomplish this, review a condition and then raise the exception if the condition turns out to be true.
A raised exception generally alerts users or the calling application. To manually throw out Python exceptions, you have to use the keyword “raise”. From there, you can include a message explaining the exception as well.
For instance, a developer might want a user to enter a date in an application. The date chosen by the user must be the current day or sometime in the future. The program should raise an exception if a user enters a date from the past.
Using Assertions
Using assertion is a different method to raise an exception. When using this method, you declare that a condition is true before you run a statement.
Next, the statement runs if the condition checks out as true. Then, the control goes on to the following statement. The program issues an AssertionError if the condition turns out not to be true.
Syntax Errors vs. Exceptions
When you program in Python, there are two kinds of unwanted conditions you might run into. One is a syntax error and the other is an exception.
Syntax error exceptions happen if the code doesn’t work with keywords in Python. The code must also fit naming styles and programming structures to avoid syntax errors.
If the interpreter catches an invalid syntax in the parsing stage, it presents a “SyntaxError” exception. At this point, the program halts and fails at the place where the syntax error occurred. For this reason, you can’t handle syntax errors.
It’s also important to note that Python issues the “TypeError” exception if it finds incorrect types of data. Other built-in exception examples include ModuleNotFoundError, ImportError, SystemError, MemoryError, and OSError.
Exceptions and Finally
The final Python error handling optional block goes by the appropriate name of “finally”. It doesn’t matter if the “try” block’s code raises an exception or not, the “finally” block runs either way.
The code in the corresponding “except” block runs when there’s an exception. After that, the “finally” block’s code runs. The code located in the “else” block runs if there aren’t any exceptions. At last, the “finally” block takes its turn to run.
Keep in mind that there might not be an “else” box in the process. Also, remember that the code in the “finally” block will always run and it’s where you should store your “clean up” codes.
Clean-up Actions
There’s an optional clause with the “try” statement that aims to define clean-up actions. You need to execute these actions no matter what the circumstance is.
If an exception happens during a try clause execution, an except clause might handle it. The exception gets raised again after the “finally” clause if it doesn’t get handled by an” except” clause.
Note that an exception might occur while executing an “else” or “except” clause. The exception gets raised again after executing the “finally” clause.
At this point, the “finally” clause performs a continue, return, or break statement. Exceptions don’t get raised again at this juncture.
The “finally” clause executes just before the break if the “try” statement arrives at a continue, return, or break statement. The returned value comes from the return statement of the “finally” clause.
Certain objects will define pre-defined clean-up actions to launch if the object isn’t needed anymore. This process happens whether the operation using the object is successful or not.
Adding Notes
After an exception gets made so that it can be raised later, it typically contains information to explain the error that happened. In some cases, it is helpful to add details after catching an exception in Python.
Because of this, Python exceptions use add_note(note) to get a series and include it in the exception’s list of notes. The standard Python traceback rendering contains every note in the precise order they got made after catching an exception.
Understanding How to Throw Exceptions
Now you know how to throw exceptions in Python and several other helpful tips. You can contact us at Programmers.io if you need to hire Python Development team. We’ve been helping businesses get to the next level since 2012.
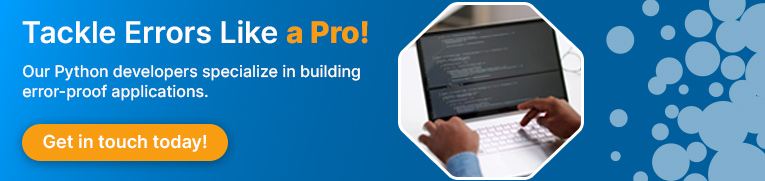
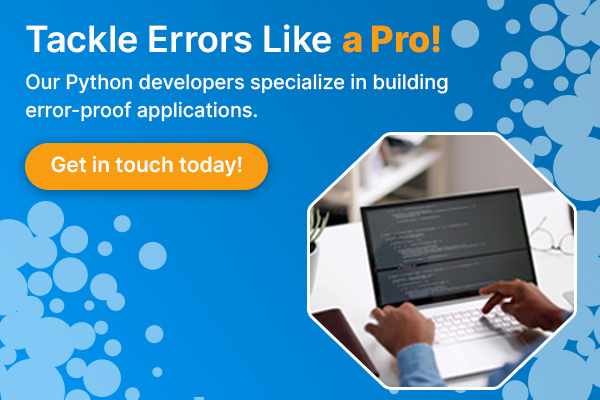
How can we help you?
We have hundreds of highly-qualified, experienced experts working in 70+ technologies.
